最近整了一个压缩文件的工具类,功能:
-
压缩文件夹,也可以压缩单独的文件
-
压缩空文件夹(额..)
-
目录结构是否保留可选
-
压缩复杂文件夹(文件夹中带有文件夹)
-
不保留目录结构时重命名同名文件
import java.io.*;
import java.util.HashMap;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
* @author 落尘
public class ZipUtils {
private static HashMap<Object, Object> index = new HashMap<>();
private static int count = 0;
public static void main(String[] args) {
try {
File file = zip("zipfile.zip", new File("C:\\TEMP\\doc"),false);
System.out.println(file.getAbsolutePath());
} catch (IOException e) {
e.printStackTrace();
* 压缩目标文件/夹, 存放于相同目录下(同级)
* @param compressedFileName 压缩后的文件名
* @param filePath 需要压缩的文件路径
* @param keepStructure 是否保留目录结构
* @return 压缩后的文件对象
* @throws IOException 压缩异常
public static File zip(String compressedFileName,String filePath,boolean keepStructure) throws IOException {
return zip(compressedFileName,new File(filePath),keepStructure);
* 压缩目标文件/夹, 存放于相同目录下(同级)
* @param compressedFileName 压缩后的文件名
* @param file 需要压缩的文件
* @param keepStructure 是否保留目录结构
* @return 压缩后的文件对象
* @throws IOException 压缩异常
public static File zip(String compressedFileName,File file,boolean keepStructure) throws IOException {
if (file == null || !file.exists()){
return null;
if (isEmpty(compressedFileName)){
compressedFileName = file.getName();
// 维护一个目录
if (keepStructure){
count = 0;
index = new HashMap<>(10);
// 定义压缩文件名称
File zipFile = new File(file.getParentFile().getAbsolutePath() + File.separator + compressedFileName);
// 压缩流对象
ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream(zipFile));
// 文件直接压缩,文件夹递归压缩
if (!file.isDirectory()){
addCompressedFile(zipOut,null,file);
}else {
addCompressedFloder(zipOut,null,file,keepStructure);
zipOut.close();
System.out.println("压缩完成");
return zipFile;
* 压缩文件夹
* @param zipOut 压缩流
* @param parentPath 压缩文件路径
* @param file 文件夹
* @param keepStructure 是否保留目录结构
private static void addCompressedFloder(ZipOutputStream zipOut,String parentPath,File file,boolean keepStructure) throws IOException {
if (file == null || !file.exists()) {
return;
parentPath = empty(parentPath);
if (!file.isDirectory()){
if (keepStructure){
addCompressedFile(zipOut,parentPath,file);
}else{
addCompressedFile(zipOut,null,file);
}else{
System.out.println("读取目标文件夹"+file.getName());
File[] files = file.listFiles();
assert files != null;
// 生成空文件夹
if (keepStructure) {
zipOut.putNextEntry(new ZipEntry(parentPath + file.getName() + File.separator));
for (File openFile: files) {
addCompressedFloder(zipOut,parentPath + file.getName() + File.separator,openFile,keepStructure);
* 压缩文件
* @param zipOut 压缩流
* @param parentPath 压缩文件目录
* @param file 文件夹
* @throws IOException
private static void addCompressedFile(ZipOutputStream zipOut,String parentPath,File file) throws IOException {
InputStream input = new FileInputStream(file);
String fileName = file.getName();
System.out.print("压缩目标文件"+fileName);
if (parentPath != null){
System.out.println(" 目录:" + parentPath + fileName);
zipOut.putNextEntry(new ZipEntry(parentPath + fileName));
} else {
System.out.println(" 目录:" + fileName);
if (index.containsKey(fileName)){
count ++;
zipOut.putNextEntry(new ZipEntry("(" + count + ")" + fileName));
index.put("(" + count + ")" + fileName,true);
} else {
zipOut.putNextEntry(new ZipEntry(fileName));
index.put(fileName,true);
int temp;
while((temp=input.read())!=-1){
zipOut.write(temp);
zipOut.closeEntry();
input.close();
private static String empty(String s){
if (isEmpty(s)){
return "";
return s;
private static boolean isEmpty(String str) {
return str == null || "".equals(str.trim());
压缩单个文件
压缩文件夹
- 保留目录结构
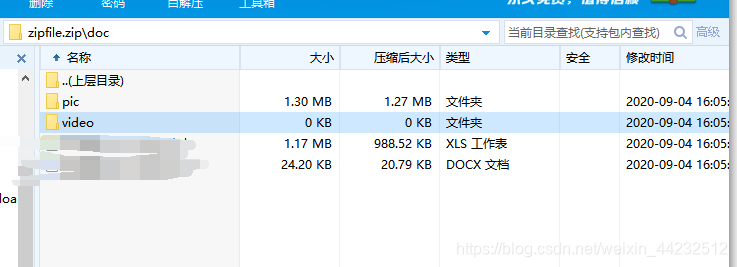
- 不保留目录结构
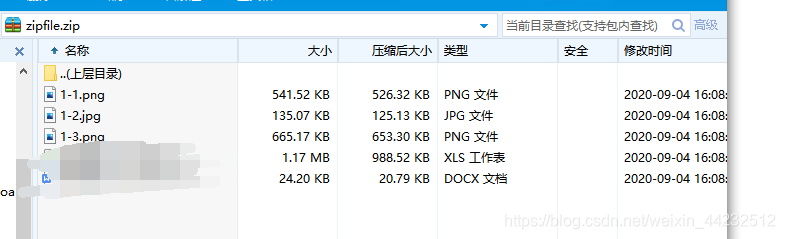
压缩复杂文件夹
简单的测试...
压缩复杂文件夹不保留目录结构时同名文件的处理
对于同名文件处理是在前面加个数字,保留目录结构时:
这边是维护了一个hashmap作为目录保证文件名的唯一,有更好的做法欢迎评论留言一起讨论
1、打开一个或者多个*.class文件,XJad反编译后,重命名为*.java文件,
保存至当前文件夹,并在编辑器中打开查看;
2、打开一个文件夹,XJad将该文件夹下所有*.class文件进行反编译,并保存至该文件夹下,
依据包路径信息生成文件夹路径,如com.spring.framework.*,
将建立com\spring\framework的文件夹结构;
3、打开一个*.jar文件,XJad将该Jar文件中的所有*.class文件解压缩到临时目录并进行反编译,
并将源文件带包路径信息保存至当前文件夹下名称为“~” + *.jar 的文件夹中;
当你需要修改一些文件的名称时,可以参考该文章。
例如:在你利用收集表等方法收集的图片时(此时文件的名称是包括提交者的姓名的),你需要将收集的图片的名称修改成统一的格式(例如:姓名+学号 等等)。
========
1、XJad是基于Jad核心的Java源程序反编译软件,内置Jad1.5.8e2;
2、可处理多个*.class文件,可以处理文件夹内的所有文件,甚至可以处理*.jar文件;
3、带有多页面文本编辑器,也可集成在资源管理器中,随时点击右键都可进行操作;
4、支持java语法的高亮显示;
使用说明:
========
1、打开一个或者多个*.class文件,XJad反编译后,重命名为*.java文件,
保存至当前文件夹,并在编辑器中打开查看;
2、打开一个文件夹,XJad将该文件夹下所有*.class文件进行反编译,并保存至该文件夹下,
依据包路径信息生成文件夹路径,如com.spring.framework.*,
将建立com\spring\framework的文件夹结构;
3、打开一个*.jar文件,XJad将该Jar文件中的所有*.class文件解压缩到临时目录并进行反编译,
并将源文件带包路径信息保存至当前文件夹下名称为“~” + *.jar 的文件夹中;
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.9</version>
</dependency>
import org.apache.commons.lang3.StringUtils;
import org.slf
public static void zipFile(String filePath) {
Log.e("开始压缩文件", System.currentTimeMillis() + "");
Stri.
db 里边存了一堆允许重名的文档,需要导出,且自动给重名的文档叠加数字尾缀。比如有文档名字分别是【全国富婆通讯录】、【全国富婆通讯录】、【全国富婆通讯录(1)】...
java.util.zip.ZipException: duplicate entry
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
* 文件操作
* Created by heavenick on 2015/7/8.
public class FileUtil...
23 final File file = newFile(targetFilePath);4 if (!file.exists()) {5 file.mkdirs();6 }7 RandomAccessFile randomAccessFile = null;8 IInArchive inArchive = null;910 ...
* @return net.ourway.cf3.CallResult
@RequestMapping(value = "/toPendingTopicZip", method = RequestMethod.GET, produces =