SVG 是使用 XML 来描述二维图形和绘图程序的语言。
-
SVG 指可伸缩矢量图形 (Scalable Vector Graphics)
-
SVG 用来定义用于网络的基于矢量的图形
-
SVG 使用 XML 格式定义图形
-
SVG 图像在放大或改变尺寸的情况下其图形质量不会有所损失
-
SVG 是万维网联盟的标准
-
SVG 与诸如 DOM 和 XSL 之类的 W3C 标准是一个整体
SVG文件包含的形状如下:
-
矩形 <rect>
矩形包含起始点坐标以及宽高信息。
id="rect3713"
width="29.666916"
height="27.528765"
x="28.865108"
y="77.838989"
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1" />
-
圆形 <circle>
圆形包含中心点坐标以及半径。
<circle
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1"
id="path5986"
cx="88.198929"
cy="92.27153"
r="13.630746" />
- 椭圆 <ellipse>
椭圆包含中心点坐标以及XY轴半径,圆形可以认为是特殊的椭圆,即XY轴半径相同。
<ellipse
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1"
id="path5984"
cx="64.946495"
cy="121.13665"
rx="14.165285"
ry="7.2162771" />
x1="36.883194" y1="142.51821" x2="69.490076" y2="142.51821"
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1" />
- 折线 <polyline>
折线包含多个点,每个点包含XY轴坐标。
<polyline
points="20,20 40,25 60,40 80,120 120,140 200,180"
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1" />
- 多边形 <polygon>
多边形可以认为是封闭的折线。
<polygon
points="100,10 150,190 60,210"
style="fill:none;fill-opacity:1;fill-rule:evenodd;stroke:#000000;stroke-width:0.2;stroke-miterlimit:4;stroke-dasharray:none;stroke-opacity:1" />
- 路径 <path>
路径是使用命令的形式记录形状。
style="fill:none;stroke:#000000;stroke-width:0.26458332px;stroke-linecap:butt;stroke-linejoin:miter;stroke-opacity:1"
d="M 102.09696,131.02562 151.27455,113.6531"
id="path5994"
用于路径的命令如下:
M = moveto
L = lineto
H = horizontal lineto
V = vertical lineto
C = curveto
S = smooth curveto
Q = quadratic Belzier curve
T = smooth quadratic Belzier curveto
A = elliptical Arc
Z = closepath
注释:以上所有命令均允许小写字母。大写表示绝对定位,小写表示相对定位。
以上形状的效果如下:
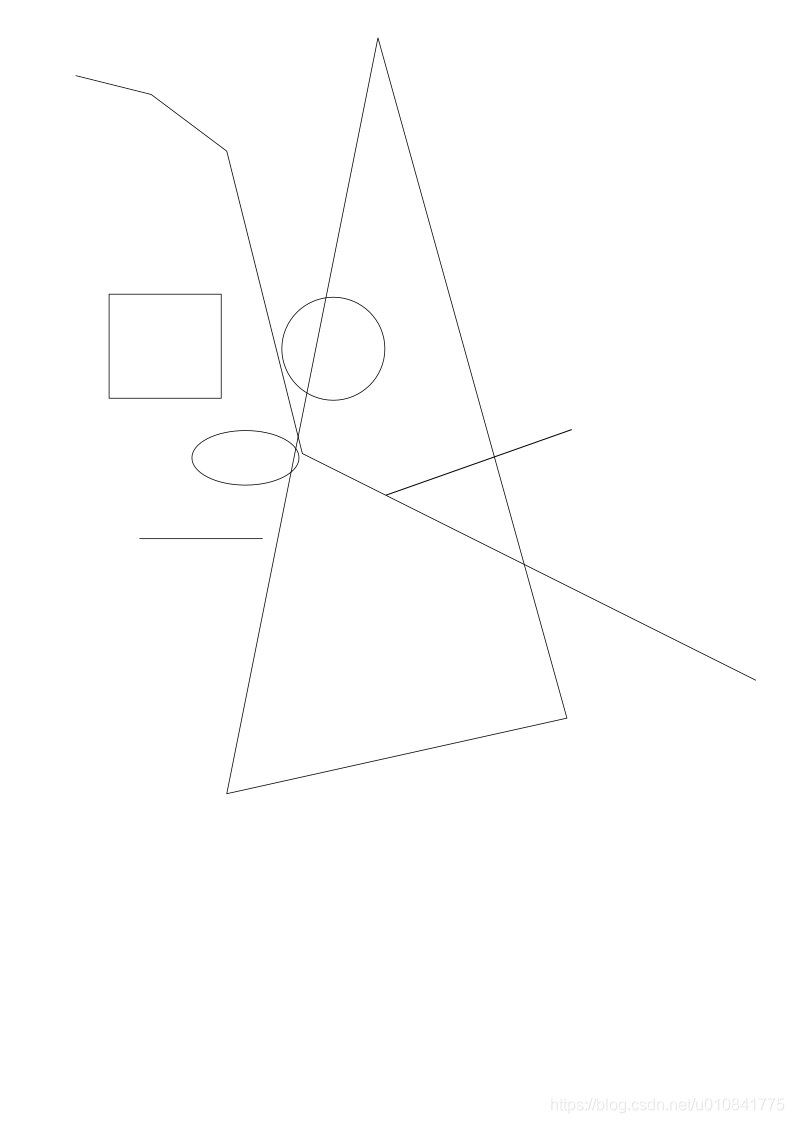
SVG文件基于xml,针对xml解析的python库较多,这里采用ElementTree进行解析。
Element 对象的常用属性:
- tag:string 对象,表示数据代表的种类
- attrib:dictionary 对象,表示附有的属性
- text:string 对象,表示 element 的内容
- child elements:若干子元素
Element 对象的常用方法: - iter(tag=None):遍历该 Element 所有后代,也可以指定 tag 进行遍历寻找
- findall(match):查找当前元素下 tag 或 path 能够匹配的直系节点
- find(match):查找当前元素下 tag 或 path 能够匹配的首个直系节点
- get(key, default=None):获取元素指定 key 对应的属性值,如果没有该属性,则返回default值。
解析流程如下:
将SVG文件传入ElementTree模块,获取xml树根。
先获取SVG图形宽高。
对xml树枝进行遍历,符合SVG图形的树枝,对于图形参数进行解析。
主函数如下:
def parser(svg_file):
tree = ET.parse(svg_file)
root = tree.getroot()
width = root.get('width')
height = root.get('height')
if len(width) == 0 or len(height) == 0:
print "width or height is 0"
exit(1)
if "mm" in width:
width = width[:-2]
if "mm" in height:
height = height[:-2]
print width, height
for elem in root.iter():
try:
_, tag_suffix = elem.tag.split('}')
except ValueError:
continue
if tag_suffix in svg_shapes:
tag_suffix = tag_suffix.capitalize()
print tag_suffix
shape_class = globals()[tag_suffix](elem)
图形解析以矩形为例,需要获取矩形的起始点及长宽:
class Rect:
def __init__(self, xml_node):
self.xml_node = xml_node
if self.xml_node is not None:
rect_el = self.xml_node
self.x = float(rect_el.get('x')) if rect_el.get('x') else 0
self.y = float(rect_el.get('y')) if rect_el.get('y') else 0
self.rx = float(rect_el.get('rx')) if rect_el.get('rx') else 0
self.ry = float(rect_el.get('ry')) if rect_el.get('ry') else 0
self.width = float(rect_el.get('width')) if rect_el.get('width') else 0
self.height = float(rect_el.get('height')) if rect_el.get('height') else 0
print("Rect: x=%f, y=%f, rx=%f, ry=%f, width=%f, height=%f"
% (self.x, self.y, self.rx, self.ry, self.width, self.height))
else:
self.x = self.y = self.rx = self.ry = self.width = self.height = 0
print("Rect: Unable to get the attributes for %s", self.xml_node)
完整代码及SVG文件可从github下载:
https://github.com/ai2robot/svg_parser
#SVG到JSON一种将SVG字符串转换为JSON数据并可选地呈现统计信息的方法。
#Usage创建新实例,提供svg字符串,还可以选择传入options 。
var svg_json = new SVGToJSON ( svg [ , options ] ) ;
#API ## Object.json SVGToJSON()返回一个对象。 OBJ.json是主要的JSON数据。 它是所有SVG标签的数组。
var svg_json = new SVGToJSON ( svg ) ;
svg_json = {
json : [ ... ]
## Object.json [I]中的每个标签Object.json有四个值。
var first_tag = svg_json . json [ 0 ] ;
first_tag = {
attrs : {
# 打开文件
tree = etree.ElementTree(file=r'/home/260190/PycharmProjects/auto_svgtopdf/test.svg') # 保证每次操作均为原始model文件
root = tree.getroot()
元素查找方法
一、match为tag;不含嵌套,返回第一个/所有匹配的Element;可查找特定属性attrib[@id=“title1”]
文章目录1.SVG概述2.复数3.svgpathtools功能4.基本用法4.1.构造函数4.2.Path编辑4.3.读SVG4.4.写SVG4.5.point函数
1.SVG概述
SVG是一种图形文件格式,它的英文全称为Scalable Vector Graphics,意思为可缩放的矢量图形。它是基于XML(Extensible Markup Language),由World Wide Web Consortium(W3C)联盟进行开发的。严格来说应该是一种开放标准的矢量图形语言,可让你设计激动人心的、高
以下代码需要nodejs环境,以及安装request-promise-native库,其实就是调用一个接口,任何语言环境都可以实现
const reqPromise = require('request-promise-native')
const api = 'https://service-bk2c1l6z-1251215691.ap-beijing.apigateway.myqc...
注意:这个项目几乎已经死了。 但是,如果您喜欢这个想法,请尝试联系我,我们会实现它。 干杯!
SVG l10n 助手
这个小软件主要面向翻译和处理SVG文件的 l10n 团队。 这可能对图表、信息图表、图表等有用。
主要(未来)功能包括:
将SVG文件解析为XML并识别 l10n 字符串
隔离字符串并创建某种目录,类似于 PO 文件
为翻译人员提供工作GUI ,可能类似于 poedit
根据目录文件创建无限数量的不同翻译
将翻译导出回SVG文件
软件依赖:
Python 2.7
gettext(尚未实现)
任何 .po 文件编辑器(我推荐 poedit)
所需的 Python 模块:
xml文件
elementtree(现在都在测试中,只有一个会存活下来)
pprint(可选,仅用于测试目的)
当然,让自动化工具翻译一些字符串可能会导
/* 创建RsvgHandle对象 */
handle = rsvg_handle_new_from_file(argv[1], &error);
if (error != NULL) {
printf("Failed to load SVG file: %s\n", error->message);
g_error_free(error);
return 1;
/* 获取SVG图像的尺寸 */
rsvg_handle_get_dimensions(handle, &dimension);
/* 创建cairo_surface_t对象 */
cairo_surface_t *surface = cairo_image_surface_create(CAIRO_FORMAT_ARGB32, dimension.width, dimension.height);
cairo_t *cr = cairo_create(surface);
/* 渲染SVG图像 */
rsvg_handle_render_cairo(handle, cr);
/* 保存图像到文件 */
cairo_surface_write_to_png(surface, "output.png");
/* 释放资源 */
cairo_destroy(cr);
cairo_surface_destroy(surface);
g_object_unref(handle);
return 0;
上述程序首先创建了一个RsvgHandle对象,该对象表示要解析的SVG文件。然后使用rsvg_handle_get_dimensions函数获取SVG图像的尺寸。接下来,创建了一个cairo_surface_t对象,该对象表示图像的绘制区域。然后使用rsvg_handle_render_cairo函数将SVG图像渲染到cairo_surface_t对象上。最后,使用cairo_surface_write_to_png函数将图像保存到文件中。最后,释放资源。